Don't Repeat Yourself (DRY) with Jinja2
Put a page name in a Jinja2 template file and share it everywhere.
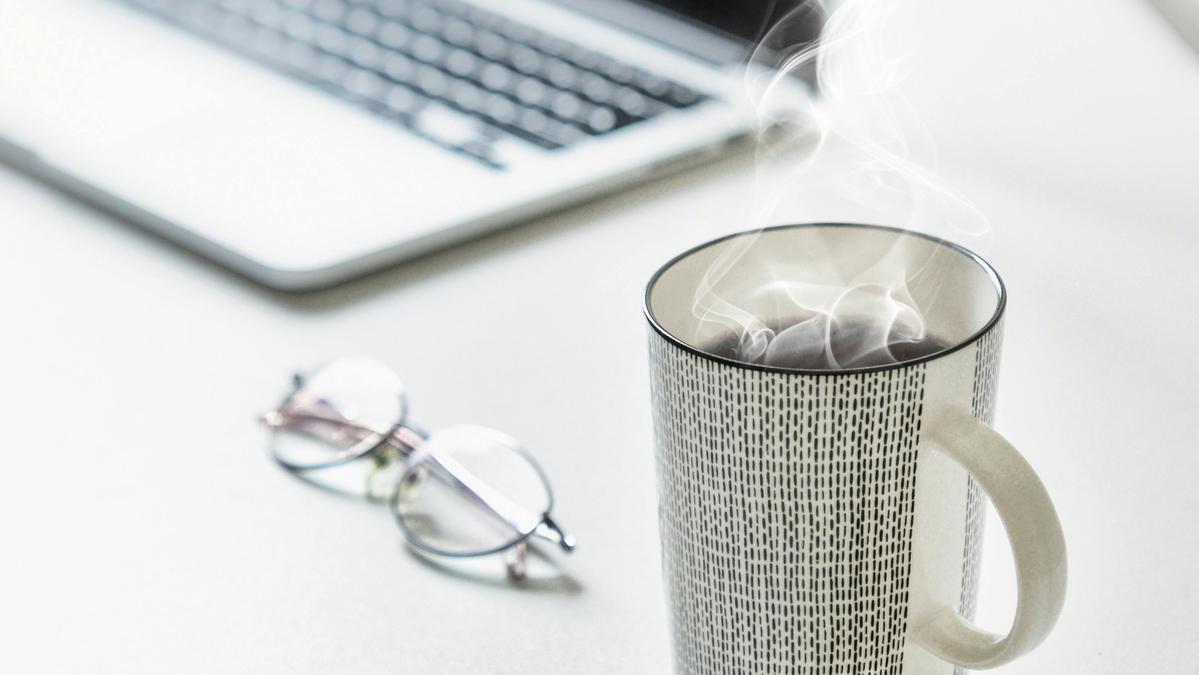
I was trying some things with Jinja2, created a small Flask app, and thought why not share this. What I wanted to achieve was to put all page names in one template file.
As with Python, when writing a lot of code, you must be careful not to repeat yourself with Jinja2. Before you know it, you end up with many template files containing the same types of information. And when you want to change something, you must edit all these template files.
This post contains all code of a basic Flask app:
- Four pages, one with a form
- Two column layout
- Header with navigation
- Uses Bootstrap (cannot live without it ...)
In case you want to try, there are only two files you need to create. I used Jinja2's DictLoader to put the templates in a Python file.
As always I do this on Ubuntu 22.04.
Extend, extend, extend
After writing some code, you want to see the page. You create the template. You add a header, footer, title, menu, etc. Do not do this! Only the output of your endpoint should be on this template!
Below is shown what we are going to achieve. The FAQ TEMPLATE is only containing 'faq data', and nothing else!
FAQ
endpoint
|
| faq
| data
v
+-----------------------------------+
| FAQ TEMPLATE |
| |
| {% extends 'page_content.html' %} |
| |
| {%- block page_data -%} |
| ... |
| faq data |
| ... |
| {%- endblock -%} |
+-----------------------------------+
|
|
| page right column
| data +-- data
| |
v v
+---------------------------------------------+
| PAGE CONTENT TEMPLATE |
| |
| {% extends 'base.html' %} |
| |
| {%- block page_content -%} |
| ... |
| {% block page_data %}{% endblock %} |
| ... |
| right column data |
| ... |
| {%- endblock -%} |
+---------------------------------------------+
|
| page content page header
| data +-- html
| |
v v
+---------------------------------------------+
| BASE TEMPLATE |
| |
| {% include 'page_header.html' %} |
| ... |
| {% block page_content %}{% endblock %} |
| ... |
+---------------------------------------------+
|
v
rendered page
Global variables in Jinja2
We can pass global variables, or data structures, to our templates in several ways:
Python code - Flask's app.config
Example:
app.config['MY_GLOBAL_VAR_KEY'] = 'my_global_var_value'
Python code - Jinja2 Environment.globals
Example:
app.jinja_env.globals['MY_GLOBAL_VAR_KEY'] = 'my_global_var_value'
Python code - Jinja2 Context processor
Example:
@app.context_processor
def inject_data():
data = {}
data['MY_GLOBAL_VAR_KEY'] = 'my_global_var_value'
return data
Jinja2 code - A Jinja2 template file with variables
This solution requires that we include this template when we need one or more variables. The advantage is that we can have all page names in a single Jinja2 template file.
Example:
{%- set global_vars = {
'MY_GLOBAL_VAR_KEY': 'my_global_var_value',
} -&}
Using page_ids
Your endpoint functions can output page specifics like a page name. But you also use page names in the navigation of the site. And we do not want to repeat ourselves!
We solve this by creating a single Jinja2 template file that holds information about our pages, including the page names. To reference page information, we use a page_id. And we use a Jinja2 macro to extract the information we want.
Running the app
Create a virtual environment and install Flask and WTForms:
pip install flask
pip install flask-wtf
Here is the tree of the directories and files. For this Flask app I use the Jinja2 DictLoader: All templates are in factory.py which means you only need two create two files!
.
├── project
│ ├── app
│ │ ├── templates (all templates are in factory.py)
│ │ │ ├── pages
│ │ │ │ ├── contact_form.html
│ │ │ │ ├── contact_form_received.html
│ │ │ │ ├── faq.html
│ │ │ │ └── home.html
│ │ │ ├── shared
│ │ │ │ ├── page_vars.html
│ │ │ │ └── page_macros.html
│ │ │ ├── base.html
│ │ │ ├── page_content.html
│ │ │ └── page_header.html
│ │ └── factory.py
│ └── run.py
The code
We have two Python files:
- run.py, in the 'project' directory
- factory.py, in the 'app' directory
# run.py
from app.factory import create_app
host = '127.0.0.1'
port = 5050
app = create_app()
app.config['SERVER_NAME'] = host + ':' + str(port)
if __name__ == '__main__':
app.run(
host=host,
port=port,
use_debugger=True,
use_reloader=True,
)
# factory.py
from flask import current_app, Flask, redirect, render_template, url_for
from flask_wtf import FlaskForm
from jinja2 import Environment, DictLoader, FileSystemLoader
from wtforms import StringField, SubmitField
from wtforms.validators import DataRequired, Length
class ContactForm(FlaskForm):
name = StringField(
'Name',
validators=[DataRequired(), Length(min=2, max=40)],
)
submit = SubmitField('Next')
def create_app():
app = Flask(__name__, instance_relative_config=True)
app.config['SECRET_KEY'] = 'Your secret key'
app.config["TEMPLATES_AUTO_RELOAD"] = True
# set some global variables
app.jinja_env.globals['site_info'] = {
'name': 'My site',
}
# use a dict instead of file system
app.jinja_loader = DictLoader(get_templates_dict())
@app.route('/')
def home():
return render_template(
'pages/home.html',
page_id='home',
)
@app.route('/faq')
def faq():
faqs = {
'How to make a list?': 'Use a dictionary.',
'How to get an answer?': 'Contact us.',
}
return render_template(
'pages/faq.html',
page_id='faq',
faqs=faqs,
)
@app.route('/contact', methods=['GET', 'POST'])
def contact():
form = ContactForm()
if form.validate_on_submit():
name = form.name.data
return redirect(url_for('contact_form_received', name=name))
return render_template(
'pages/contact.html',
page_id='contact',
form=form,
)
@app.route('/contact-form-received/<name>')
def contact_form_received(name):
return render_template(
'pages/contact_form_received.html',
page_id='contact_form_received',
name=name,
)
@app.context_processor
def inject_data():
current_app.logger.debug('()')
data = {}
right_column_blocks = {
'news': {
'title': 'News',
'text': 'This is some news text ...',
},
'most_viewed': {
'title': 'Most viewed',
'text': 'This is some most viewed text ...',
},
}
data['right_column_blocks'] = right_column_blocks
return data
return app
# here are the 'files' in the templates directory.
# remove the Jinja2 DictLoader if you use the filesystem
def get_templates_dict():
return {
'base.html': """
{# base.html #}
{%- from 'shared/page_macros.html' import page_title -%}
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>{{ site_info['name'] }} | {{ page_title(page_id) }}</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-T3c6CoIi6uLrA9TneNEoa7RxnatzjcDSCmG1MXxSR1GAsXEV/Dwwykc2MPK8M2HN" crossorigin="anonymous">
</head>
<body>
{% include 'page_header.html' %}
<div class="container mt-3" id="main">
<div class="row">
<div class="col">
{% block page_content %}{% endblock %}
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-C6RzsynM9kWDrMNeT87bh95OGNyZPhcTNXj1NW7RuBCsyN/o0jlpcV8Qyq46cDfL" crossorigin="anonymous"></script>
</body>
</html>""",
'page_content.html': """
{# page_content.html #}
{%- from 'shared/page_vars.html' import page_vars as pv -%}
{%- from 'shared/page_macros.html' import page_title -%}
{% extends 'base.html' %}
{% block page_content %}
<div class="row">
<div class="col-12 mb-3 col-md-8 border-end">
<h1>
{{ page_title(page_id) }}
</h1>
{% block page_data %}{% endblock %}
</div>
<div class="col-12 mb-3 col-md-4">
{%- for column_block_id, column_block_data in right_column_blocks.items() -%}
<div class="row">
<div class="col">
<h2>
{{ column_block_data['title'] }}
</h2>
<p>
{{ column_block_data['text'] }}
</p>
</div>
</div>
{%- endfor -%}
</div>
</div>
{% endblock %}""",
'page_header.html': """
{# page_header.html #}
{%- from 'shared/page_macros.html' import topnav_menu_item -%}
<nav class="navbar navbar-expand-md bg-body-tertiary">
<div class="container">
<a class="navbar-brand" href="{{ url_for('home') }}">
{{ site_info['name'] }}
</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav me-auto">
{{ topnav_menu_item(page_id) }}
</ul>
</div>
</div>
</nav>""",
'shared/page_macros.html': """
{# shared/page_macros.html #}
{%- from 'shared/page_vars.html' import page_vars as pv -%}
{%- macro page_title(page_id) -%}
{%- if page_id in pv.page_id_infos -%}
{{ pv.page_id_infos[page_id]['title'] }}
{%- else -%}
{{ '?' }}
{%- endif -%}
{%- endmacro -%}
{%- macro topnav_menu_item(page_id) -%}
{%- for menu_item_page_id, actives in pv.topnav_menu_item_page_id_actives.items() -%}
{%- set menu_item = pv.page_id_infos[menu_item_page_id] -%}
{%- set menu_item_title = menu_item['title'] -%}
{%- set menu_item_url = url_for(menu_item['endpoint']) -%}
{%- set active = '' -%}
{%- if page_id in actives -%}
{%- set active = 'active' -%}
{%- endif -%}
<li class="nav-item">
<a class="nav-link {{ active }}" href="{{ menu_item_url }}">
{{ menu_item_title }}
</a>
</li>
{%- endfor -%}
{%- endmacro -%}""",
'shared/page_vars.html': """
{# shared/page_vars.html #}
{%- set page_vars = {
'page_id_infos': {
'home': {
'title': 'Home',
'endpoint': 'home',
},
'faq': {
'title': 'FAQ',
'endpoint': 'faq',
},
'contact': {
'title': 'Contact',
'endpoint': 'contact',
},
'contact_form_received': {
'title': 'Contact form received',
'endpoint': 'contact_form_received',
},
},
'topnav_menu_item_page_id_actives': {
'home': ['home'],
'faq': ['faq'],
'contact': ['contact', 'contact_form_received'],
},
} -%}""",
'pages/home.html': """
{# pages/home.html #}
{% extends 'page_content.html' %}
{% block page_data %}
<p>
Welcome
</p>
<p>
Your config:
</p>
<table class="table table-sm">
<thead>
<tr><th>Key</th><th>Value</th></tr>
</thead>
<tbody>
{%- for k, v in config.items() -%}
<tr><td>{{ k }}</td><td>{{ v }}</td></tr>
{%- endfor -%}
</tbody>
</table>
{% endblock %}""",
'pages/faq.html': """
{# pages/faq.html #}
{% extends 'page_content.html' %}
{%- block page_data -%}
<ul>
{%- for question, answer in faqs.items() -%}
<li>
Q: {{ question }}
<br>
A: {{ answer }}
</li>
{%- endfor -%}
</ul>
{%- endblock -%}""",
'pages/contact.html': """
{# pages/contact.html #}
{% extends 'page_content.html' %}
{%- block page_data -%}
<form method="POST">
{{ form.csrf_token }}
<div class="mb-3">
<label for="form-field-name" class="form-label">
{{ form.name.label }}
</label>
{{ form.name(size=20, class_='form-control') }}
</div>
{% if form.name.errors %}
<ul class="text-danger">
{% for error in form.name.errors %}
<li>
{{ error }}
</li>
{% endfor %}
</ul>
{% endif %}
{{ form.submit(class_='btn btn-primary') }}
</form>
{%- endblock -%}""",
'pages/contact_form_received.html': """
{# pages/contact_form_received.html #}
{% extends 'page_content.html' %}
{%- block page_data -%}
<p>
Thank you {{ name }}
</p>
{%- endblock -%}""",
}
To run, go to the project directory and type:
python run.py
Then point your browser to:
127.0.0.1:5050
Summary
We do not want to repeat ourselves and want the name of a page in only one Jinja2 template file. We solved this by using a 'page_id', that is used everywhere to retrieve the page name / title from a global variables dictionary in a Jinja2 template file.
The dictionary must be imported by template files where we want to access this information. This is just one of the options to store global variables.
Recent
- Hiding database UUID primary keys of your web application
- Don't Repeat Yourself (DRY) with Jinja2
- SQLAlchemy, PostgreSQL, maximum number of rows per user
- Show the values in SQLAlchemy dynamic filters
- Secure data transfer with Public Key encryption and pyNaCl
- rqlite: a high-availability and distributed SQLite alternative
Most viewed
- Using Python's pyOpenSSL to verify SSL certificates downloaded from a host
- Using UUIDs instead of Integer Autoincrement Primary Keys with SQLAlchemy and MariaDb
- Connect to a service on a Docker host from a Docker container
- Using PyInstaller and Cython to create a Python executable
- SQLAlchemy: Using Cascade Deletes to delete related objects
- Flask RESTful API request parameter validation with Marshmallow schemas